Implementing Graph Algorithms Using DSA in Python
Implementing Graph Algorithms Using DSA in Python
Introduction
Graph algorithms play a pivotal role in solving a wide range of real-world problems, from network analysis to social media recommendation systems. As essential components of data structures and algorithms (DSA), mastering graph algorithms is crucial for any programmer looking to excel in problem-solving. While traditionally associated with languages like Java, Python has emerged as a popular choice for implementing graph algorithms due to its simplicity and versatility. In this blog post, we’ll delve into the world of graph algorithms and explore how DSA with Python can be used to implement them efficiently.
Understanding Graphs and Graph Algorithms
Before diving into the implementation details, let’s first understand what graphs are and why they are important. In computer science, a graph is a collection of nodes (vertices) and edges (connections) that establish relationships between these nodes. Graphs can model various real-world scenarios, such as social networks, road networks, and computer networks, making them a fundamental data structure in computer science.
Graph algorithms are procedures or techniques used to traverse, search, and analyze graphs to solve specific problems. These algorithms are essential for tasks such as finding shortest paths, detecting cycles, and identifying connected components within a graph. By mastering graph algorithms, programmers can develop efficient solutions to a wide range of graph-related problems.
Transitioning from DSA with Java to DSA with Python
Historically, Java has been the go-to language for implementing graph algorithms due to its performance and extensive standard library support. However, the verbosity and boilerplate code associated with Java can sometimes hinder the development process, especially for beginners or those looking for a more agile programming experience. This is where Python shines.
Python’s concise syntax, dynamic typing, and high-level abstractions make it an excellent choice for implementing graph algorithms. Moreover, Python’s extensive ecosystem of libraries and frameworks simplifies the implementation of complex algorithms, allowing programmers to focus more on problem-solving rather than low-level details.
Key Graph Algorithms in Python
Let’s explore some essential graph algorithms and how they can be implemented efficiently using Python:
- Breadth-First Search (BFS): BFS is a fundamental graph traversal algorithm used to explore a graph’s vertices level by level. It starts at a specified source vertex and explores all its neighbors before moving on to the next level. BFS is commonly used for tasks such as finding the shortest path in an unweighted graph and detecting connected components.
- Depth-First Search (DFS): DFS is another graph traversal algorithm that explores as far as possible along each branch before backtracking. It is often used to traverse or search for specific elements within a graph, such as finding connected components, topological sorting, and detecting cycles.
- Dijkstra’s Algorithm: Dijkstra’s algorithm is a popular algorithm for finding the shortest paths between nodes in a weighted graph. It works by iteratively selecting the vertex with the smallest tentative distance from the source vertex and updating the distances to its neighbors accordingly. Dijkstra’s algorithm is widely used in applications such as routing protocols and network optimization.
- Minimum Spanning Tree (MST) Algorithms: MST algorithms are used to find the minimum spanning tree of a graph, which is a subgraph that connects all the vertices with the minimum possible total edge weight. Algorithms such as Kruskal’s algorithm and Prim’s algorithm are commonly used to find the MST of a graph efficiently.
Implementing Graph Algorithms in Python
Now, let’s see how these graph algorithms can be implemented using Python:
“`python
Breadth-First Search (BFS) Implementation
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
while queue:
vertex = queue.popleft()
if vertex not in visited:
print(vertex)
visited.add(vertex)
queue.extend(graph[vertex] – visited)
Depth-First Search (DFS) Implementation
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start)
for next_vertex in graph[start] – visited:
dfs(graph, next_vertex, visited)
Dijkstra’s Algorithm Implementation
import heapq
def dijkstra(graph, start):
distances = {vertex: float(‘infinity’) for vertex in graph}
distances[start] = 0
queue = [(0, start)]
while queue:
current_distance, current_vertex = heapq.heappop(queue)
if current_distance > distances[current_vertex]:
continue
for neighbor, weight in graph[current_vertex].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(queue, (distance, neighbor))
return distances
Minimum Spanning Tree (MST) – Kruskal’s Algorithm Implementation
class DisjointSet:
def __init__(self):
self.parent = {}
self.rank = {}
def make_set(self, vertex):
self.parent[vertex] = vertex
self.rank[vertex] = 0
def find(self, vertex):
if self.parent[vertex] != vertex:
self.parent[vertex] = self.find(self.parent[vertex])
return self.parent[vertex]
def union(self, vertex1, vertex2):
root1 = self.find(vertex1)
root2 = self.find(vertex2)
if root1 != root2:
if self.rank[root1] > self.rank[root2]:
self.parent[root2] = root1
else:
self.parent[root1] = root2
if self.rank[root1] == self.rank[root2]:
self.rank[root2] += 1
def kruskal_mst(graph):
mst = set()
disjoint_set = DisjointSet()
for vertex in graph[‘vertices’]:
disjoint_set.make_set(vertex)
edges = list(graph[‘edges’])
edges.sort()
for edge in edges:
weight, vertex1, vertex2 = edge
if disjoint_set.find(vertex1) != disjoint_set.find(vertex2):
mst.add(edge)
disjoint_set.union(vertex1, vertex2)
return mst
Example Usage
graph = {
‘A’: {‘B’: 1, ‘C’: 4},
‘B’: {‘A’: 1, ‘C’: 2, ‘D’: 5},
‘C’: {‘A’: 4, ‘B’: 2, ‘D’: 1},
‘D’: {‘B’: 5, ‘C’: 1}
}
print(“BFS Traversal:”)
bfs(graph, ‘A’)
print(“DFS Traversal:”)
dfs(graph, ‘A’)
print(“Shortest distances from source vertex using Dijkstra’s Algorithm:”)
print(dijkstra(graph, ‘A’))
print(“Minimum Spanning Tree using Kruskal’s Algorithm:”)
print(kruskal_mst(graph))
“`
conclusion
implementing graph algorithms using DSA with Java offers a powerful combination that enables programmers to solve complex problems efficiently. By leveraging Python’s simplicity, elegance, and extensive library support, programmers can focus on the logic and efficiency of their solutions rather than getting bogged down by language-specific complexities. Moreover, Python’s popularity and community support ensure that resources, tutorials, and libraries for DSA are readily available, making it an excellent choice for implementing graph algorithms.
Whether you’re a beginner learning the ropes of DSA or an experienced programmer looking to enhance your problem-solving skills, DSA with Python opens up a world of possibilities in the realm of graph algorithms. So, dive into the world of DSA with Python, and unlock your potential as a proficient problem solver in the fascinating domain of graphs and algorithms.
Also Read – One of the key features of Araya Health Centre
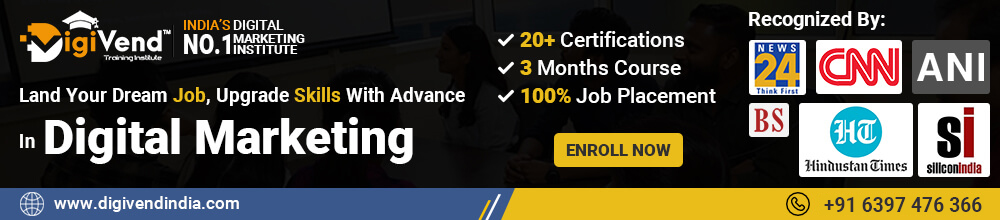